- Published on
How to reset mocks in Jest with practical code examples
- Authors
- Name
- Bipin Parajuli
- @bipinprjl
How to reset mocks in Jest with practical code examples
Jest is a popular JavaScript testing framework that provides an easy and effective way to test your code. One of the features of Jest is the ability to create and use mock functions. Mock functions are functions that replace the implementation of a real function with a fake implementation that you can control and observe during your tests.
However, sometimes we need to reset the mock functions between tests. In this blog post, we'll explore how to reset mocks in Jest and provide practical code examples.
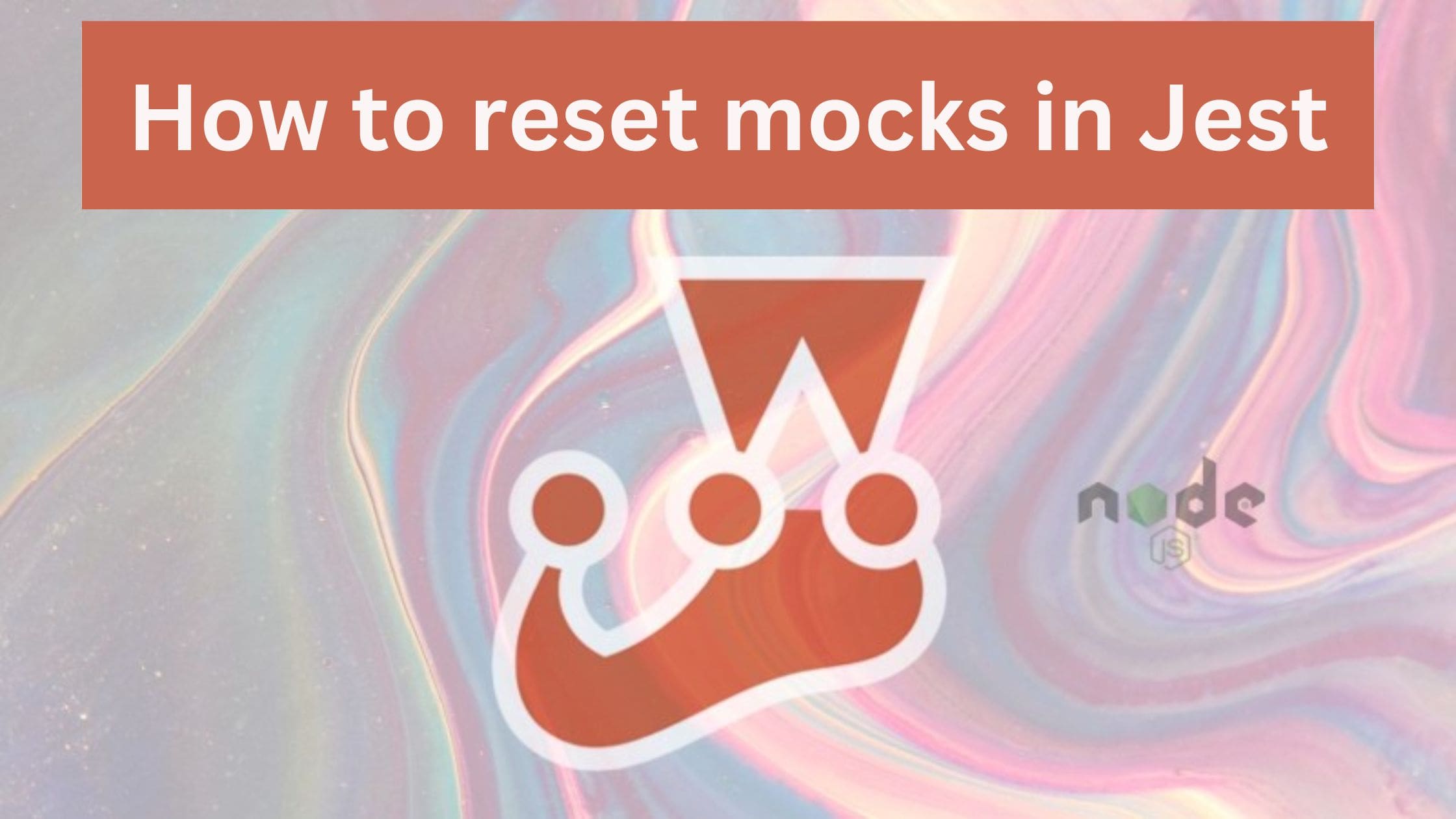
Table of contents
Introduction
Prequirities
Mocking function
Why Reset Mocks in Jest?
How to Reset Mocks in Jest
Resetting All Mocks Globally
Resetting a Specific Mock Function
Resetting Mock Implementation
Best practice for resetting mock
Conclusion
Prequirities
- Basic understanding of JavaScript
- Familiarity with the Jest testing framework
- Understanding of mock functions and how to use them in Jest tests.
Mocking Functions
In Jest, mocks are used to simulate the behavior of real objects. This can be useful for testing code that depends on external APIs or other libraries. In jest, we can create a mock function simply with jest.fn(). The jest mock function allows us to capture calls, return values, and change implementation. However, it's important to reset mocks between tests so that they don't affect each other. Let’s get started by creating a simple mock function.
//mocking a function
const myFn = jest.fn()
test('first test', () => {
myFn()
expect(myFn).toHaveBeenCalledTimes(1)
})
In the above code example jest.fn() creates a mock function that returns undefined by default. With jest expect calls of mock function are tested.
Why reset mock in jest
When using mock functions in Jest, the mock function retains the history of its calls, return values, and arguments. This can be useful in certain cases, such as when you need to assert that a function was called a specific number of times or with specific arguments. However, in other cases, you may want to reset the mock function's history between tests to ensure that each test is isolated and not influenced by the previous test's results.
For example, imagine you have two tests that both call the same mock function:
//mocking a function
const myFn = jest.fn()
test('first test', () => {
myFn()
expect(myFn).toHaveBeenCalledTimes(1)
})
test('second test', () => {
myFn()
expect(myFn).toHaveBeenCalledTimes(1)
})
In this example, the first test calls myFn() once and expects it to have been called once. The second test also calls myFn() again and expects it also to have been called once. However, because the mocks are not reset between tests, the second test actually expects myFn() to have been called two times. So the above test case failed and throw an error that the mock function has been called for two times but the test expects it to be called only one time.
To fix this, we can use reset mocks before running the second test. By resetting all mocks to their initial state, the second test will expect myFn() to have been called only once.
How to reset mock in Jest
There are two ways to reset mocks in jest.
- Resetting mocks globally
- Resetting specific mock function
Resetting mocks globally
Jest provides a jest.resetAllMocks() function that resets all mock functions' history. This function is useful when you want to reset all mock functions globally between tests. Let’s reset all mocks globally in the above test so that both test cases will pass.
//mocking a function
const myFn = jest.fn()
beforeEach(() => {
jest.resetAllMocks()
})
test('first test', () => {
myFn()
expect(myFn).toHaveBeenCalledTimes(1)
})
test('second test', () => {
myFn()
expect(myFn).toHaveBeenCalledTimes(1)
})
If we run the above test with the command yarn test or npm test both tests were passed.
we use the beforeEach() function to reset all mock functions before each test. This ensures that each test is isolated and not influenced by the previous test's results.
Resetting a specific mock
Alternative to resetting the mock globally we can also reset a specific mock by using the mockReset() method of jest. This function is useful when you want to reset a specific mock function between tests.
//mocking a function
const myFn = jest.fn()
test('first test', () => {
myFn()
expect(myFn).toHaveBeenCalledTimes(1)
myFn.mockReset()
})
test('second test', () => {
myFn()
expect(myFn).toHaveBeenCalledTimes(1)
})
In this example, we use the mockFn.mockReset() function to reset the mockFn mock function between tests. This ensures that each test is isolated and not influenced by the previous test's results.
Resetting mock implementation
In addition to resetting the mock function's history, Jest also provides a way to reset the mock function's implementation. This can be useful when you want to change the mock function's behavior between tests.
To reset a mock function's implementation, you can use the mockFn.mockImplementation() function with no arguments. This will reset the mock function's implementation to its default behavior.
Best practices for resetting mocks
Here are some best practices for resetting mocks in Jest:
- Always reset mocks between tests. This will ensure that each test is isolated from the others and that the mocks are reset to their initial state before each test.
- Reset mocks if you change the behavior of a mock between tests. This will ensure that the new behavior is only applied to the current test and that previous tests are not affected.
- Use mockFn.mockClear() if you only need to clear a single mock. This will ensure that the function is called as if it were a real function each time.
Conclusion
Resetting the mock object in Jest is a relatively straightforward process and can be accomplished with ease using a few simple steps. Knowing how to reset mocks helps to ensure that your tests are running reliably and accurately, which is essential for writing test. By following the practical code examples provided above, you should now have a good understanding of how to properly reset the mock object in Jest and be well on your way toward writing more reliable tests.
You can find the full code for this blog post in this repository.